Chapter 8 Tuples
8.1 Tuples¶
Five basic types of objects have been introduced so far in this book, including the number types int, float, None type for no ouput of function, the string type for text IO and the Boolean type for control flow. Tuples are another type of objects in Python, which represents an ordered container of objects. In particular, a tuple object represents a sequence of objects, which can be heterogeneous.
A tuple literal is written as a comma-separated list of literals or identifiers, enclosed in a pair of parentheses:
>>> t=(1,2,3)
>>> t
(1, 2, 3)
>>> type(t)
<class 'tuple'>
>>> x='a'
>>> y=True
>>> u = (1.5, x, y)
>>> u
(1.5, 'a', True)
>>> type(u)
<class 'tuple'>
>>> v=()
>>> v
()
>>> type(v)
<class 'tuple'>
>>> w=(1.3,)
>>> w
(1.3 ,)
>>> type(w)
<class 'tuple'>
>>> d=(1.3)
>>> d
>>> 1.3
>>> type(d)
<type 'float'>
In the example above, t is a tuple that consists of three items, 1, 2, 3, which are all integer objects. u is a tuple that consists of 3 items, 1.5, ‘a’, and True, which belong to different types. The literal of u consists of a floating point number literal (1.5), an identifier (x) that represents a string (‘a’), and an identifier y that represents a Boolean object (True). When an identifier is used in a tuple literal, the object that the identifier is bound to is taken as the corresponding item in the tuple.
v is en empty tuple — a tuple that contains no items. w is a tuple that consists of a single item (1.3). When writing a tuple literal with a single item, a comma must be added after the literal or identifier of the item. This is to differentiate the literal of a single-item tuple from the use of parentheses in an expression, which specifies the order of evaluation. The value of d, for example, is a floating point number 1.3, because the right hand side of the assignment statement for d consists of an expression with a single floating point literal, enclosed in a pair of parentheses.
The memory structure for the example above is shown in Fig. 8.1. Each tuple consists of a sequence of object references. When an identifier is used to specify a tuple item, the real object that the identifier is bound to is added to the tuple. Hence there is no direct link between the identifiers u and x, for example, except that an item of t and x are bound to the same object ‘a’.
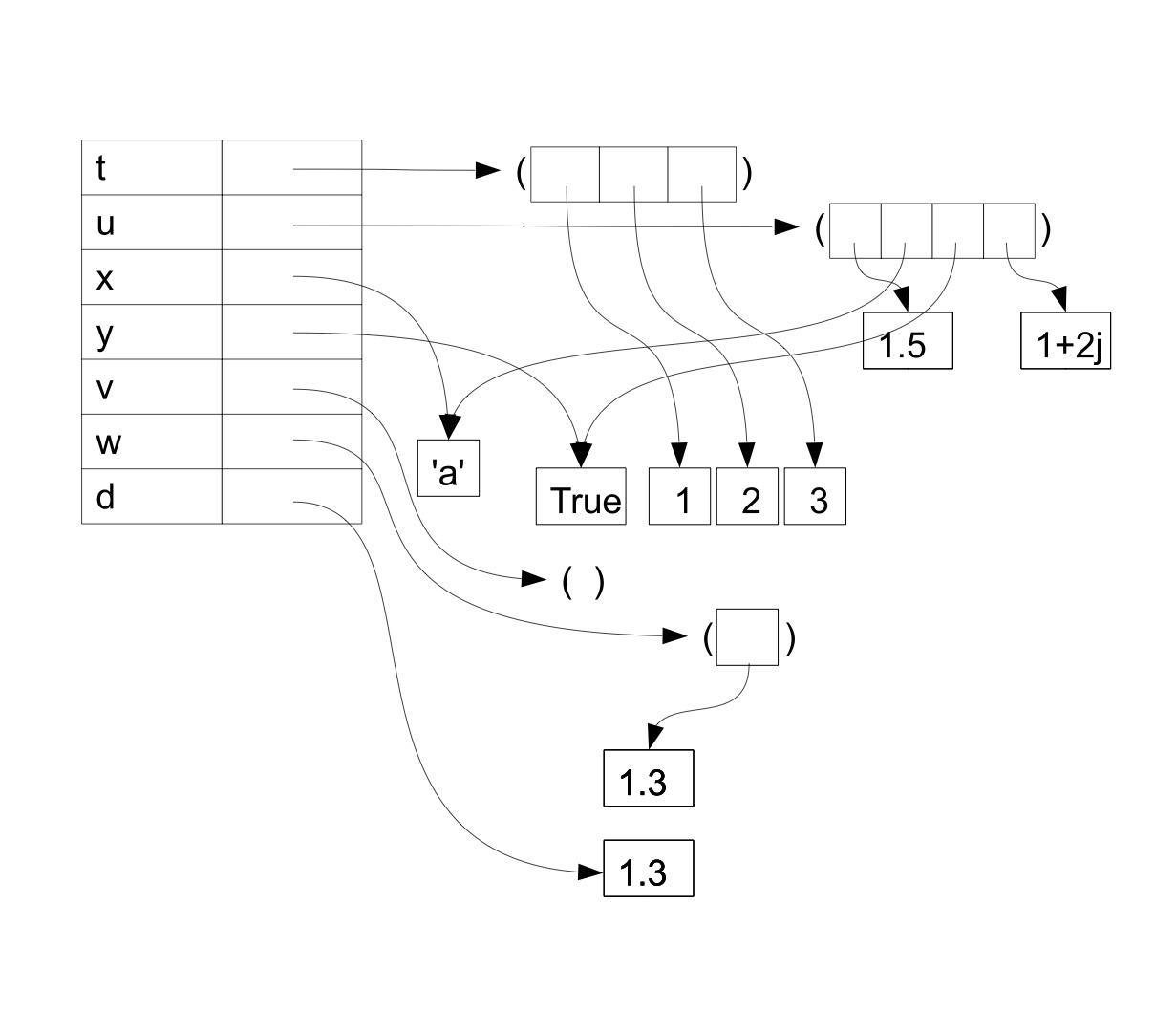
Fig. 8.1 Tuple objects in memory¶
Tuple operators. Tuples support exactly the same set of operations as strings do. In fact, since a string object represents a sequence of characters, a tuple object can be regarded as a ‘special string object’, which contains arbitrary objects rather than characters. For example, the + and * operators apply to tuples:
Similar to the case of strings, the + operator concatenates two tuple objects into a new tuple object, while the * operator makes copies of a tuple object. The memory structure of the example above is shown in Fig. 8.2.
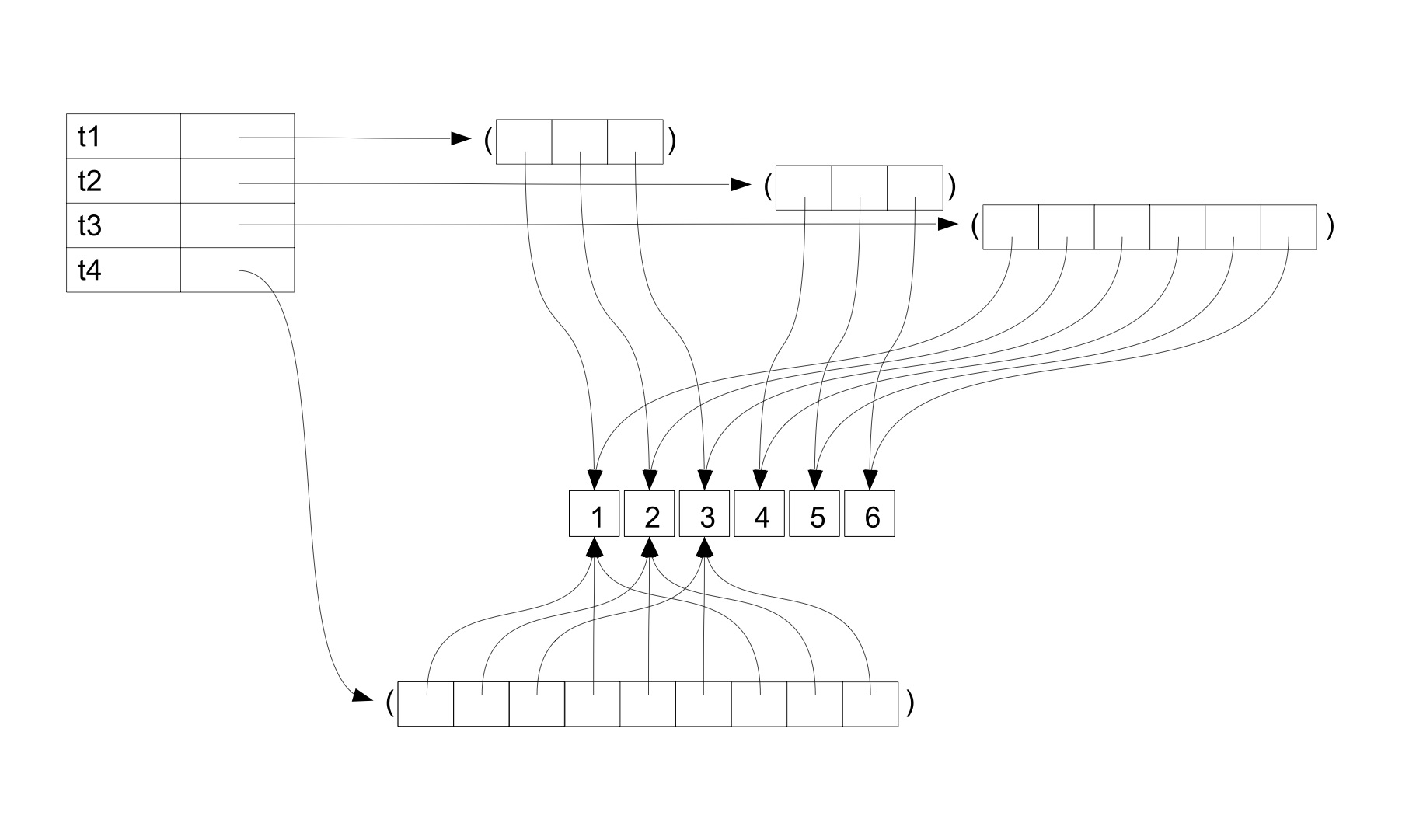
Fig. 8.2 Addition and multiplication for tuple objects¶
The getitem and getslice operations, and the len function, also apply to tuples.
>>> t = ('a', 0, 1+3j)
>>> t[0]
>>> 'a'
>>> t[1:3]
(0, (1+3j))
>>> t[-2:]
(0, (1+3j))
>>> len(t)
3
>>> len(t+t)
6
>>> len(t*3)
9
>>> len(())
0
>>> len((1,))
1
The in and not in operators can also be applied to tuples, indicating whether an object is an item in a tuple or not.
>>> t=(1,'a',True)
>>> 1 in t
True
>>> 1.0 not in t
False
>>> 'a' in t
True
>>> 'ab' in t
False
>>>
Conversion between tuples and other types. A tuple object cannot be converted to a number. However, it can be converted into a string or a Boolean type object. When a tuple is converted into a string, its literal form is taken, with each element in its own literal form. When a tuple is converted into a Boolean object, the result is False only if the tuple is empty, and True otherwise. Such conversions offer a handy way of making use of implicit conversions, such as putting tuples in print and while statements where string and Boolean objects are expected.
>>> x='a'
>>> y=True
>>> str((x,y,-0.3))
"('a', True , -0.3)"
>>> bool((1,2,3))
True
>>> bool(())
False
>>> print ((x,y,-0.3))
('a', True , -0.3)
>>> if (1,2,3):
print (True)
True
While numbers and Boolean objects cannot be converted to tuples, strings can be converted to tuples, with each character being mapped into one element in the resulting tuple object.
>>> tuple (1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
>>> tuple(True)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'bool' object is not iterable
>>> tuple('abc')
('a', 'b', 'c')
When the assignment statement was discussed earlier, it was mentioned that the left hand side of an assignment statement can be an identifier or a tuple. In the latter case, the left hand side must be a tuple of identifiers, and the right hand side must be a tuple of the same size as the left hand side. Each object in the right hand side tuple is assigned to the corresponding identifier in the left-hand-side tuple. for example,
>>> (x, y)=(1, 2)
>>> x
1
>>> y
2
>>> (x, y, z) = (3, 'a', x)
>>> x
3
>>> y
'a'
>>> z
1
When a tuple-to-tuple assignment is executed, Python first tries to find the objects that are contained in the right-hand-side tuple. In the second example above, it finds the int object 3, and string object ‘a’ and the int object 1, as referred to by the identifier x. Then Python assigns the three objects to the corresponding identifiers in the left-hand-side tuple, resulting in x being 3, y being ‘a’ and z being 1. Note that the value of x changes in the execution of this assignment statement, in a similar way to the execution of the statement x = x + 1.
Tuple assignment provides a convenient way of swapping two variables.
>>> x=1
>>> y=2
>>> (x,y)=(y,x)
>>> x
2
>>> y
1
© Copyright 2024 GS Ng.