Chapter 3 The First Python Program
3.5 The Structure of Python Programs¶
The structure of a Python program can be summarized as Fig. 3.3, where a program consists of a set of statements. Each statement can contain one or more mathematical expressions, and each expression consists of literals, identifiers and operators. Python runs a program by executing its statements, each of which fulfills a specific functionality.
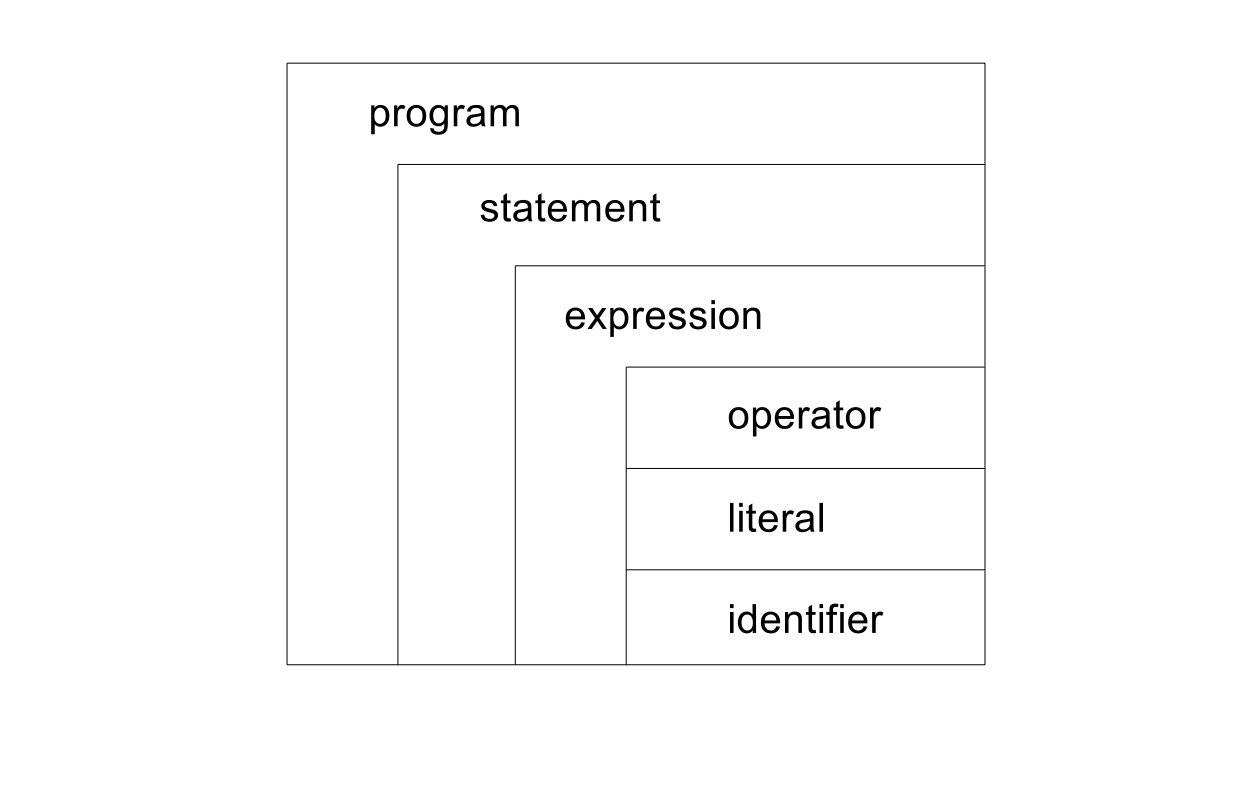
Fig. 3.3 The structure of a Python program¶
As mentioned earlier, all statements are executed in a fixed and mechanical way. Take the assignment statement for example. The right hand side of = must be an expression, and the left hand side must be an identifier. The statement is executed by evaluating the expression first, and then binding the resulting object with associating the left-hand-side identifier. Understanding the execution of each type of statement is crucial to the understanding and design of Python programs. A list of the statements that have been introduced up to this section is shown in Table 3.1.
Statement |
Comment |
---|---|
assignment |
<identifier>=<expression>, <identifier>+=<expression>, |
import |
Import module |
Text output |
Note that an expression is sometimes used as a command by itself. For example, by typing ‘3+5’ in IDLE, one obtains the result ‘8’. However, as stated earlier, the usage above is possible only because IDLE displays the value of an expression by default. In a standalone Python program, this is not possible.
Each expression is evaluated into a single object during program execution. An expression is evaluated by applying operators to operands, which can be literals or identifiers. Operators are applied according to a fixed precedence, and parentheses can be used to explicitly specify the order of evaluation. Table 3.2 give a summary of all the operators that have been introduced, which include arithmetic operators (+, -, * etc.), the dot (.) operator, the indexing operator ([]) and the function call operator (()). Their precedence is as follows. The dot, indexing and function call operators have higher precedence than the arithmetic operators, while ** has higher precedence than *, /, // and % in arithmetic operators. Operators with the same precedence are executed from left to right.
Operator |
Comment |
---|---|
+, −, ∗, /, //, %, ∗∗ |
Arithmetic operators |
. |
Load content from module |
[] |
getitem, getslice |
() |
Function call |
Both identifiers and literals represent Python objects in the underlying implementation. Each Python object is associated with a type. Two categories of types have been introduced in this book, including numbers (int, float, long, complex) and strings. While numbers are essential for mathematical computation, strings are useful for text input and output functionalities. A list of the types that have been introduced and their corresponding literals is shown in Table 3.3. More types will be introduced later.
Type |
Literal |
Comment |
---|---|---|
int |
1, 0, -1 |
Integer |
long |
1L |
Implicit large integer |
float |
3.5, 5e-1 |
Floating point number |
complex |
1 - 3j |
Complex numbers |
str |
‘abc’, “1 !” |
For input output |
Comments. In addition to statements, a Python program can also consist of comments. Comments are not executed when a Python program is executed, but they are useful for making Python programs more readable. It is a good habit to write comments when programming, since proper comments can not only help other programmers understand the design of a program, but also remind a programmer of her own thinking when designing the program. Comments are particularly important in large software projects.
Python comments start with a # symbol. In a line of Python code, all the texts after the first # symbol are treated as comments, and ignored during execution.
In the example above, the first line contains some comments that explain the purpose of the identifier g. The comments are useful for the understanding of subsequent uses of the identifier. The second line in the example above illustrates another use of comments, which is to temporarily delete a part of Python code. In this example, the value of y is 9.81, rather than 10.81, since the text ‘+1’ is placed after a # symbol. In this case, the programmer might have started with the statement y = g + 1, but then decided to try y = x instead. She, however, does not want to completely remove the ‘+1’ part, since there is a chance that she wants to add it back after some testing. Therefore, she chooses to keep ‘+1’ in the line but as a comment. In programming terminology, she commented out the part of code. The last line prints 9.81 on the console.
Comments can also be written as a single line, with the # symbol at the beginning of the line. This form of comment is called in-line comment. In-line comments are typically used to explain the design of a set of multiple statements under the comment line.
Combined Statements. Multiple Python statements can be written in a single line, separated by semi-colons (;). Such combinations offer compactness in code, but can make programs more difficult to understand.
Check your understanding
- To tell the computer what you mean in your program.
- Comments are ignored by the computer.
- For the people who are reading your code to know, in natural language, what the program is doing.
- The computer ignores comments. It’s for the humans that will “consume” your program.
- Nothing, they are extraneous information that is not needed.
- Comments can provide much needed information for anyone reading the program.
- Nothing in a short program. They are only needed for really large programs.
- Even small programs benefit from comments.
3.5Q-1: What are comments for?
© Copyright 2024 GS Ng.