Chapter 4 Functions
4.1 Function Definition¶
As discussed before, many mathematical functions cannot be computed by using a simple expression. There are cases where the value of a custom function must be obtained by iterative numerical computation. In such cases, the calculation of a return value requires a procedure that consists of multiple statements, typically representing a dynamic control flow.
Instead, Python provides a special statement, the def statement, for the definition of functions that consist of multiple statements. To begin with, consider the following function f:
The syntax of the def statement is illustrated in Fig. 4.1. The first line of a def statement starts with the def keyword, followed by a custom function name, and a comma-separated list of parameters, enclosed in a pair of parenthesess, and finally a colon. After the def line is an indented statement block, which is called the function body. The function body contains one or many return statements, which begin with the return keyword, followed by an expression:
return <expression >
The def statement performs two tasks. First, it defines a function object. Second, it associates the function object with the function name given in the def line.
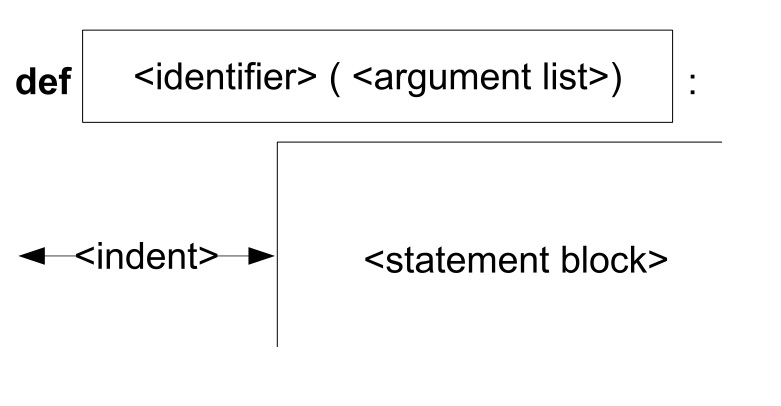
Fig. 4.1 The def statement¶
In a function call, the function name is used as the identifier for accessing the function object. The parameters defined in the def line are assigned the argument values given in the function call expression, before the function body is executed. During the execution of the function body, if one return statement is executed, the execution of the function body is terminated, with the value of the expression in the return statement being taken as the return value of the function call.
In the example above, the def statement defines a function object with two parameters, and assigns the name f to it. When the call f(1,2) is evaluated, the argument 1 and 2 are assigned to the parameters x and y, respectively, and the function body is executed when the return statement is executed, the function call returns, with the value of x + z, which is 5.
If no return statements are executed before the whole function body is executed, a special return value, None, is taken as the return value of the function call. Here None belongs to a new type, the NoneType. It is the only object that belongs to the type.
The object None is equal to neither 0, nor ‘’, nor False, because NoneType is a different type as compared to a number, the string or the Boolean type. None can be converted into a string, ‘None’, or a Boolean object, False.
In the example above, when the value of None is given to IDLE (i.e. by typing the identifier a directly as an expression in IDLE), nothing is shown on the console. This is different from other objects, for which IDLE display the value. By default, IDLE does not display the None value. However, when given to the print statement, None is first converted into a string, and then shown.
None serves as a special value in Python, representing the meaning ‘nothing’.
Correlated to the None object is a statement that does nothing: the pass statement. The syntax of the pass statement consists of the pass keyword only. It serves as a placeholder where a statement block is required, but nothing needs to be performed.
A simplest function can be one that takes no argument, and performs nothing:
In the example above, the function call f() does not perform anything: the function body is a single pass statement. In this example, the pass statement is necessary, because an indented statement block is required in the definition of a function. Since no return statement is executed during the execution of the function body, the return value of the function call f () is None. Note that when f () is used as a single-line expression, nothing is shown as the value of the expression. This is because IDLE shows no output for the value None, as illustrated earlier.
For a more complex example, consider a function that shows the string ‘Hello, world’ on the console.
In the example above, when the assignment statement a = g() is executed, the expression g() is evaluated first. It is a function call expression, which leads to the invoking of the function body of g. As a result, the string ‘Hello, world’ is printed to the console. The value of the function call expression g() is assigned to the identifier a. Since no return statement is executed, the default return value, None, is returned.
Check your understanding
- A named sequence of statements.
- Yes, a function is a named sequence of statements.
- Any sequence of statements.
- While functions contain sequences of statements, not all sequences of statements are considered functions.
- A mathematical expression that calculates a value.
- While some functions do calculate values, the python idea of a function is slightly different from the mathematical idea of a function in that not all functions calculate values. Consider, for example, the turtle functions in this section. They made the turtle draw a specific shape, rather than calculating a value.
- A statement of the form x = 5 + 4.
- This statement is called an assignment statement. It assigns the value on the right (9), to the name on the left (x).
4.1Q-1: What is a function in Python?
- To improve the speed of execution
- Functions have little effect on how fast the program runs.
- To help the programmer organize programs into chunks that match how they think about the solution to the problem.
- While functions are not required, they help the programmer better think about the solution by organizing pieces of the solution into logical chunks that can be reused.
- All Python programs must be written using functions
- In the first several chapters, you have seen many examples of Python programs written without the use of functions. While writing and using functions is desirable and essential for good programming style as your programs get longer, it is not required.
- To calculate values.
- Not all functions calculate values.
4.1Q-2: What is one main purpose of a function?
- def drawCircle(t):
- A function may take zero or more parameters. It does not have to have two. In this case the size of the circle might be specified in the body of the function.
- def drawCircle:
- A function needs to specify its parameters in its header.
- drawCircle(t, sz):
- A function definition needs to include the keyword def.
- def drawCircle(t, sz)
- A function definition header must end in a colon (:).
4.1Q-3: Which of the following is a valid function header (first line of a function definition)?
- def drawSquare(t, sz)
- This line is the complete function header (except for the semi-colon) which includes the name as well as several other components.
- drawSquare
- Yes, the name of the function is given after the keyword def and before the list of parameters.
- drawSquare(t, sz)
- This includes the function name and its parameters
- Make turtle t draw a square with side sz.
- This is a comment stating what the function does.
4.1Q-4: What is the name of the following function?
def drawSquare(t, sz):
"""Make turtle t draw a square of with side sz."""
for i in range(4):
t.forward(sz)
t.left(90)
- i
- i is a variable used inside of the function, but not a parameter, which is passed in to the function.
- t
- t is only one of the parameters to this function.
- t, sz
- Yes, the function specifies two parameters: t and sz.
- t, sz, i
- the parameters include only those variables whose values that the function expects to receive as input. They are specified in the header of the function.
4.1Q-5: What are the parameters of the following function?
def drawSquare(t, sz):
"""Make turtle t draw a square of with side sz."""
for i in range(4):
t.forward(sz)
t.left(90)
- def drawSquare(t, sz)
- No, t and sz are the names of the formal parameters to this function. When the function is called, it requires actual values to be passed in.
- drawSquare
- A function call always requires parentheses after the name of the function.
- drawSquare(10)
- This function takes two parameters (arguments)
- drawSquare(alex, 10):
- A colon is only required in a function definition. It will cause an error with a function call.
- drawSquare(alex, 10)
- Since alex was already defined and 10 is a value, we have passed in two correct values for this function.
4.1Q-6: Considering the function below, which of the following statements correctly invokes, or calls, this function (i.e., causes it to run)? Assume that there is a turtle named alex.
def drawSquare(t, sz):
"""Make turtle t draw a square of with side sz."""
for i in range(4):
t.forward(sz)
t.left(90)
- True
- Yes, you can call a function multiple times by putting the call in a loop.
- False
- One of the purposes of a function is to allow you to call it more than once. Placing it in a loop allows it to executed multiple times as the body of the loop runs multiple times.
4.1Q-7: True or false: A function can be called several times by placing a function call in the body of a loop.
© Copyright 2024 GS Ng.