Chapter 5 If Statment
5.1 The Boolean Type¶
The Boolean type is designed to represent logical values; it is frequently used for control flow. Different from integers, floating point numbers and strings, for which there are an infinite number of possible literals, there are only two Boolean literals: True and False.
Boolean operators. There are three logical operators for Boolean objects, which include the binary operators and and or, and the unary operator not. The effects of the three operators are shown in Fig. 5.1. a and b is True only when both a and b are True, and False otherwise. a or b is False only when both a and b are False, and True otherwise. not a is True when a is False, and False when a is True.
>>> a=True
>>> b=False
>>> a and b
False
>>> a or b
True
>>> not a
False
>>> not b
True
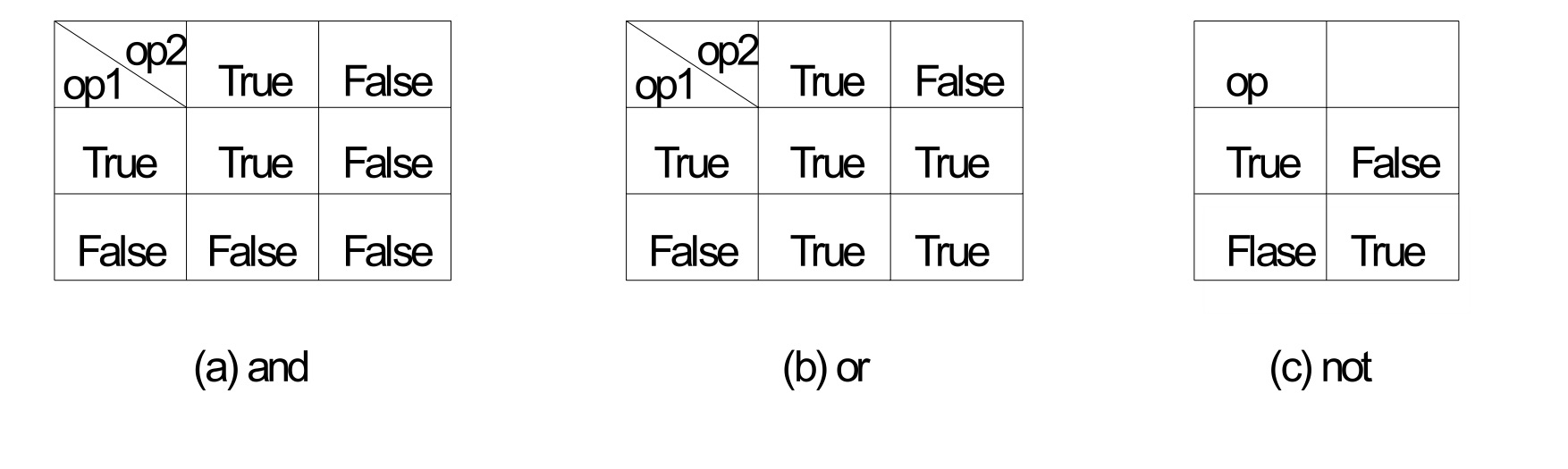
Fig. 5.1 Boolean operators. a and. b or. c not¶
Similar to arithmetic expressions, Boolean expressions can be composite, consisting of multiple Boolean objects and operators. The priority of the not operator is higher than that of and, which is in turn higher than that of or. Similar to other expressions, parentheses can be used to explicitly specify the order of evaluation in Boolean expressions.
>>> a=True
>>> b=True
>>> c=False
>>> a and not b # 'not b' first
False
>>> c and b or a # 'c' and 'b' first
True
>>> c and (b or a) # explicit
False
>>> a and b or b and c # 'a and b' -> 'b and c' -> 'or' last
True
In the last example above, a and b is evaluated first, before b and c is evaluated. The resulting values True and False are then combined by the or operator, resulting in the value True. If and and or had the same precedence, the expression would have been evaluated from left to right, resulting in the value False. With two literals and three operators, Boolean algebra is relatively simple and straightforward.
The following table summarizes the operator precedence from highest to lowest. A complete table for the entire language can be found in the Python Documentation.
Level |
Category |
Operators |
---|---|---|
7(high) |
exponent |
** |
6 |
multiplication |
*,/,//,% |
5 |
addition |
+,- |
4 |
relational |
==,!=,<=,>=,>,< |
3 |
logical |
not |
2 |
logical |
and |
1(low) |
logical |
or |
Operators resulting in Boolean values. There is a set of operators that take non-Boolean operands, yet result in Boolean values. One example of such operators is numerical comparison.
>>> 1==2 # equals
False
>>> 1!=2 # not equal
True
>>> 3>5 # greater than
False
>>> 3<5 # less than
True
>>> 3<=5 # less than or equal to
True
The == operator (i.e. =; equal) returns True if the two operands are equal, and False otherwise; the != operator (i.e. ! =; unequal) returns True if the two operands are not equal, and False otherwise; the > operator (i.e. greater than) returns True if the first operand is greater than the second operand, and False otherwise; the < operator (i.e. less than) returns True if the first operand is less than the second operator, and False otherwise; the >= operator (i.e. >=; greater than or equal to) returns True if the first operand is greater than or equal to the second operand, and False otherwise; the <= operator (i.e. <=; less than or equal to) returns True if the first operand is less than or equal to the second operand, and False otherwise. In addition to the operators above, more operators take non-Boolean operands and result in Boolean values; they will be introduced later in this book. Intuitively, the two special floating point numbers, float(‘inf’) and float(‘-inf’), which represent ∞ and −∞, respectively, conform to the rules of numerical comparison also.
>>> a=float('inf')
>>> b=float('-inf')
>>> a>10e6
True
>>> a<10
False
>>> b<-10E6
True
Multiple Boolean expressions can be combined by Boolean operators, resulting in composite Boolean expressions, which are commonly used in Python programs for control flow.
>>> a=3
>>> b=5
>>> c=2
>>> a>b and b>c
False
>>> a>b or b>c
True
>>> a>b and b>c or b>c and c<a
True
Operators that result in Boolean values (e.g. >, <) have higher precedence compared to Boolean operators (e.g. and, or). As a result, the three expressions in the example above are reduced to composite Boolean expressions after the > and < operators are evaluated. In the first two examples above, the expressions a > b and b > c are evaluated first, before the Boolean operators are evaluated. In the last example, the four expressions a > b, b > c, b > c and c > a are evaluated first, before the two and operators are evaluated. The or operator is evaluated last due to the fact that and has higher priority than or.
The operators ==, !=, <, >, <= and >= can also be cascaded, with the interpretation of logical conjunction. For example, 1 < 2 < 3 is interpreted as 1 < 2 and 2 < 3, and its value is True. Similarly, the value of 1 <= 2 < 5! = 4 is True. However, the value of 1 < 3 < 5 == 4 is False because the value of 5 == 4 is False.
Another set of operators that result in Boolean values include in and not in, which return whether a string is a sub string of another.
>>> s1 = 'bc'
>>> s2 = 'abcde'
>>> s3 = 'b'
>>> s1 in s2
True
>>> s3 not in s2
False
>>> s3 in s1
True
>>> s1 in s3
False
Yet another set of operators that result in Boolean values includes is and is not, which return whether the identifier of the object are the same.
>>> a = 123 # small number cached
>>> b = 123
>>> id(a)
19960736
>>> id(b)
19960736
>>> a is b
True
>>> a is not b
False
>>> a = 12345 # not cached
>>> b = 12345
>>> id(a)
20241576
>>> id(b)
20241408
>>> a is b
False
Type conversion from and to the Boolean type can be applied to numbers, strings and most other types to be introduced in this book.
>>> bool (0) # zero
False
>>> bool(3) # non-zero
True
>>> bool (0.0) # zero
False
>>> bool(0.1) # non-zero
True
>>> bool (1.0) # non-zero
True
>>> bool(100.0) # non-zero
True
>>> bool('') # empty
False
>>> bool('abc') # non-empty
True
>>> bool('This is a string that contains multiple characters.')
True
>>> int(True)
1
>>> int(False)
0
>>> float(True)
1.0
>>> float(False)
0.0
>>> str(True)
'True'
>>> str(False)
'False'
>>> print (False)
False
>>> print (True)
True
While the bool function converts objects of other types into Boolean values, Boolean values can be converted into other types using respective functions. In general, a number is converted to False if its value is 0, and True otherwise. A string is converted to False if it is an empty string, and True otherwise. The numeric value of False is 0, and that of True is 1. The string value of False and True are ‘False’ and ‘True’, respectively.
The and, or and not operators require Boolean operands. If non-Boolean operands are found, they are converted to Boolean values implicitly. For example, (1 and ‘a’) is True because both 1 and ‘a’ are converted to True. However, 0 is False because 0 is converted to False.
Check your understanding
- True
- True and False are both Boolean literals.
- 3 == 4
- The comparison between two numbers via == results in either True or False (in this case False), both Boolean values.
- 3 + 4
- 3 + 4 evaluates to 7, which is a number, not a Boolean value.
- 3 + 4 == 7
- 3 + 4 evaluates to 7. 7 == 7 then evaluates to True, which is a Boolean value.
- "False"
- With the double quotes surrounding it, False is interpreted as a string, not a Boolean value. If the quotes had not been included, False alone is in fact a Boolean value.
5.1Q-1: Which of the following is a Boolean expression? Select all that apply.
- x > 0 and < 5
- Each comparison must be between exactly two values. In this case the right-hand expression < 5 lacks a value on its left.
- 0 < x < 5
- This is tricky. Although most other programming languages do not allow this syntax, in Python, this syntax is allowed. However, you should not use it. Instead, make multiple comparisons by using and or or.
- x > 0 or x < 5
- Although this is legal Python syntax, the expression is incorrect. It will evaluate to true for all numbers that are either greater than 0 or less than 5. Because all numbers are either greater than 0 or less than 5, this expression will always be True.
- x > 0 and x < 5
- Yes, with an and keyword both expressions must be true so the number must be greater than 0 an less than 5 for this expression to be true.
5.1Q-2: What is the correct Python expression for checking to see if a number stored in a variable x is between 0 and 5.
- ((5*3) > 10) and ((4+6) == 11)
- Yes, * and + have higher precedence, followed by > and ==, and then the keyword "and"
- (5*(3 > 10)) and (4 + (6 == 11))
- Arithmetic operators (*, +) have higher precedence than comparison operators (>, ==)
- ((((5*3) > 10) and 4)+6) == 11
- This grouping assumes Python simply evaluates from left to right, which is incorrect. It follows the precedence listed in the table in this section.
- ((5*3) > (10 and (4+6))) == 11
- This grouping assumes that "and" has a higher precedence than ==, which is not true.
5.1Q-3: Which of the following properly expresses the precedence of operators (using parentheses) in the following expression: 5*3 > 10 and 4+6==11
© Copyright 2024 GS Ng.