Chapter 5 If Statment
5.2 Branching Using the if Statement¶
The if statement supports conditional or branching control flow. The simplest form of the if statement is shown in Fig. 5.2. It is a compound statement that consists of multiple lines. The first line contains a Boolean condition, the runtime value of which decides whether the rest of the compound statement is executed.
In particular, the first line starts with the if keyword, followed by a Boolean expression, and then a colon (:). Following the if line, a block of indented statements are written from the second line onwards. Here indentation refers to the insertion of whitespace characters, including spaces (‘’) and tabs (‘ ‘) before a statement. Common indentations include 2–8 spaces and 1–2 tabs, while spaces are preferred because different text editors can interpret a tab character as different numbers of spaces. A statement block contains one or multiple statements with equal identation.
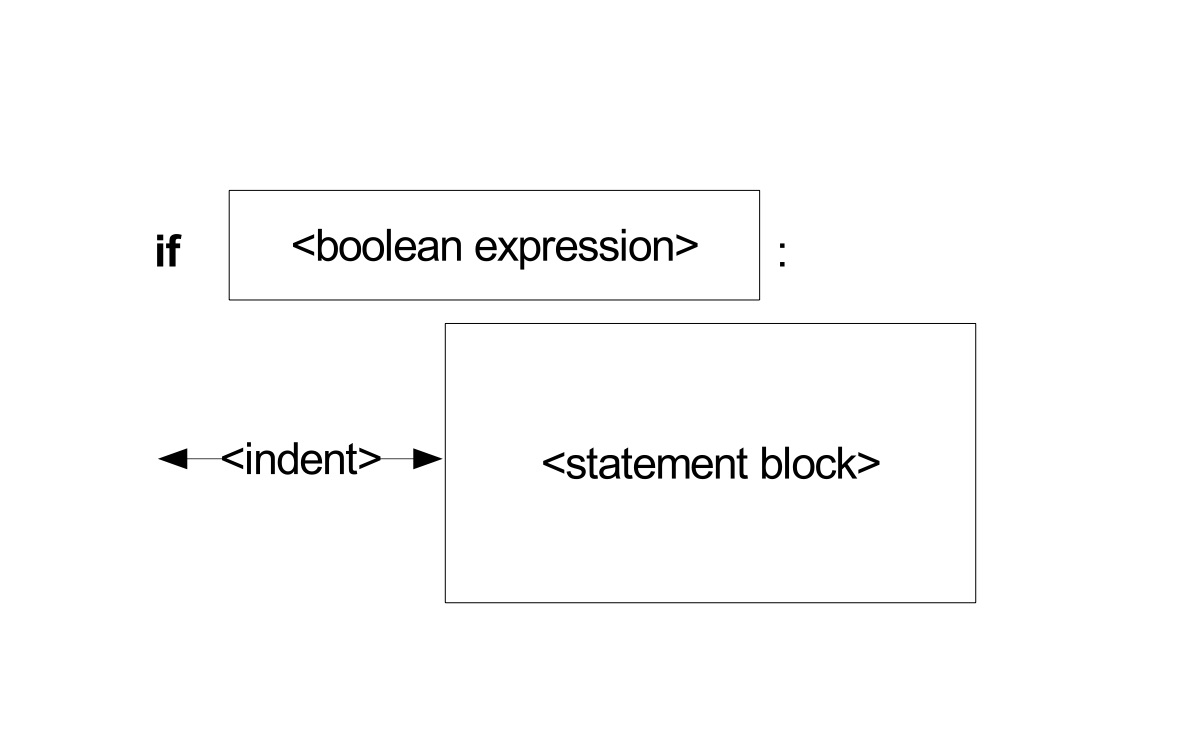
Fig. 5.2 The simplest form of the if statement.¶
Conditional execution. At runtime, the value of the Boolean expression in the if line determines whether the statement block is executed. For example,
There are two if statements in the example above. In the first if statement, the Boolean expression is a constant value True. When it is executed, the statement block under the if line is executed. In the second if statement, the Boolean expression is a constant False. When this if statement is executed, the statement block under the if line is not executed, and nothing is printed.
Below are three examples where the Boolean condition expressions are more complex than constant values.
In the first example above, the Boolean expression consists of a > operator, which takes two integer operands and returns a Boolean value. According to the values of a and b, the value of the Boolean expression is False, and hence the two statements under the if line are not executed. In the second example, the same type of Boolean expression is used, but the runtime value is True, leading to the execution of the two indented statements. The third example uses a compound Boolean expression, which is evaluated to True, leading to the execution of the statement block.
If a non-Boolean expression is put in the place of the Boolean expression in the if statement, it is automatically and implicitly converted into a Boolean value.
In the lines of code above, five values of three different types are put in the place of the Boolean expression. They are converted into the Boolean type, respectively. Again the outputs illustrate the mechanisms of Boolean conversion and conditional execution.
In a dynamic execution of the if statement, the value of the Boolean expression can be determined by user input.
The program asks the user for a number a, and prints a line if it is zero. This is achieved by using an if statement with the Boolean condition a == 0. After the compound if statement, there is a third statement in the program, which simply prints the string ‘Bye’. Python uses indentation to determine whether a statement is inside a compound statement or outside it. In this example, the last line is not indented, and therefore is not a part of the compound if statement.
The flow chat of the program is shown in Fig. 5.3(a).
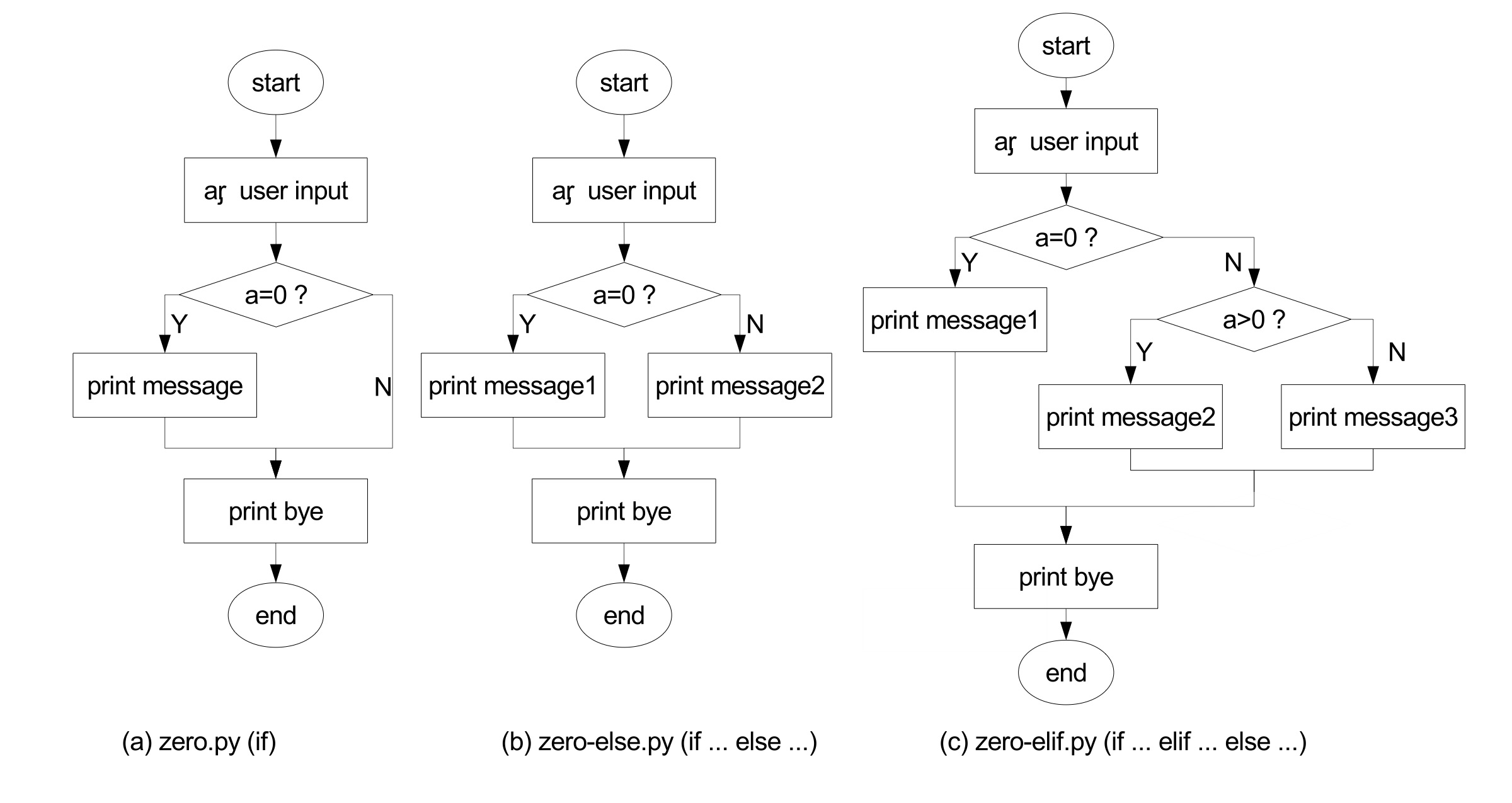
Fig. 5.3 Branching flow charts: (a) zero.py (if). (b) zero-else.py (if … else …). (c) zero-elif.py (if … elif … else …).¶
Two-way branching. zero.py gives a text response when the user input is zero, but no feedback if the user input is non-zero. In order to be more informative and also show some text response when the user input is not zero, an alternative form of the if statement can be used.
zero_else.py is a modified version of zero.py, with the if statement being extended by adding an else component, which consists of two lines. The first line is not indented; it starts with the else keyword, followed by a colon (:). The second line is a print statement, which by itself forms an indented statement block.
The if … else … structure is a single compound statement, the execution of which is controlled by the Boolean expression in the if line. If the value of the Boolean expression is True, the indented statement block under the if line is executed, but the indented statement block under the else line is not. If the value of the Boolean expression is False, the reverse happens, with the indented statement block under the else line being executed, but that under the if line being not executed. At each execution of the compound statement, only one of the two indented statement blocks is executed.
The dynamic control flow is illustrated in Fig. 5.3(b).
Multi-way branching. zero_else.py can be further extended to give different responses when the user input is positive, zero or negative, respectively. This is a three-way branching condition. However, one Boolean value can express a choice between only two options. A common solution to making a choice from more than two options is to break a multi-way condition into the concatenation of multiple two-way conditions. In this case, two Boolean conditions can be used, with the first one determining whether the user input is zero or non-zero, and the second one determining whether the user input is positive or not, if it is non-zero. The dynamic process can be illustrated by Fig. 5.3(c).
Python offers an alternative form of the if statement that allows such multi-way branching. Making use of this variation, zero_else.py can be further extended into zero_elif.py.
zero_elif.py is different from zero_else.py in that the second statement, which is a compound if… else… statement in zero_else.py, is extended into an if…elif…else… compound in zero_elif.py. The additional elif line corresponds to the additional condition in Fig. 5.3(c). It is an unindented line, starting with the keyword elif, followed by a Boolean expression, and finishing with a colon (:). Similar to the if and else cases, an indented statement block is placed under the elif line. The dynamic execution of the compound if…elif… else… statement can be illustrated with Fig. 5.3(c), where the three branches from left to right correspond to the statement blocks under the if, elif and else lines, respectively. At each execution, only one among the three branches is executed. An example of executing zero_elif.py is shown below.
> python zero_elif.py
Give me a number: 1
The number is positive
Bye
> python zero_elif.py
Give me a number: 0
The number is zero
Bye
> python zero_elif.py
Give me a number: -3
The number is negative
Bye
The following program, scoregrade.py, further demonstrates a five-way branching example. The program asks the user to enter a final-exam score, and then prints the grade (A/B/C/D) that corresponds to the score. If the score that the user entered is less than 0 or greater than 100, the program prints an error message.
The control flow diagram for this program is shown in Fig. 5.4, where the five branches from left to right correspond to the score being in the ranges [85, 100], [70, 85), [50, 70), [0, 50) and otherwise, respectively. At one execution of the program, only one branch is executed.
> python grade.py
Enter a score: 75
B
> python grade.py
Enter a score: 100
A
> python grade.py
Enter a score: 33
D
> python grade.py
Enter a score: -10
Invalid score entered
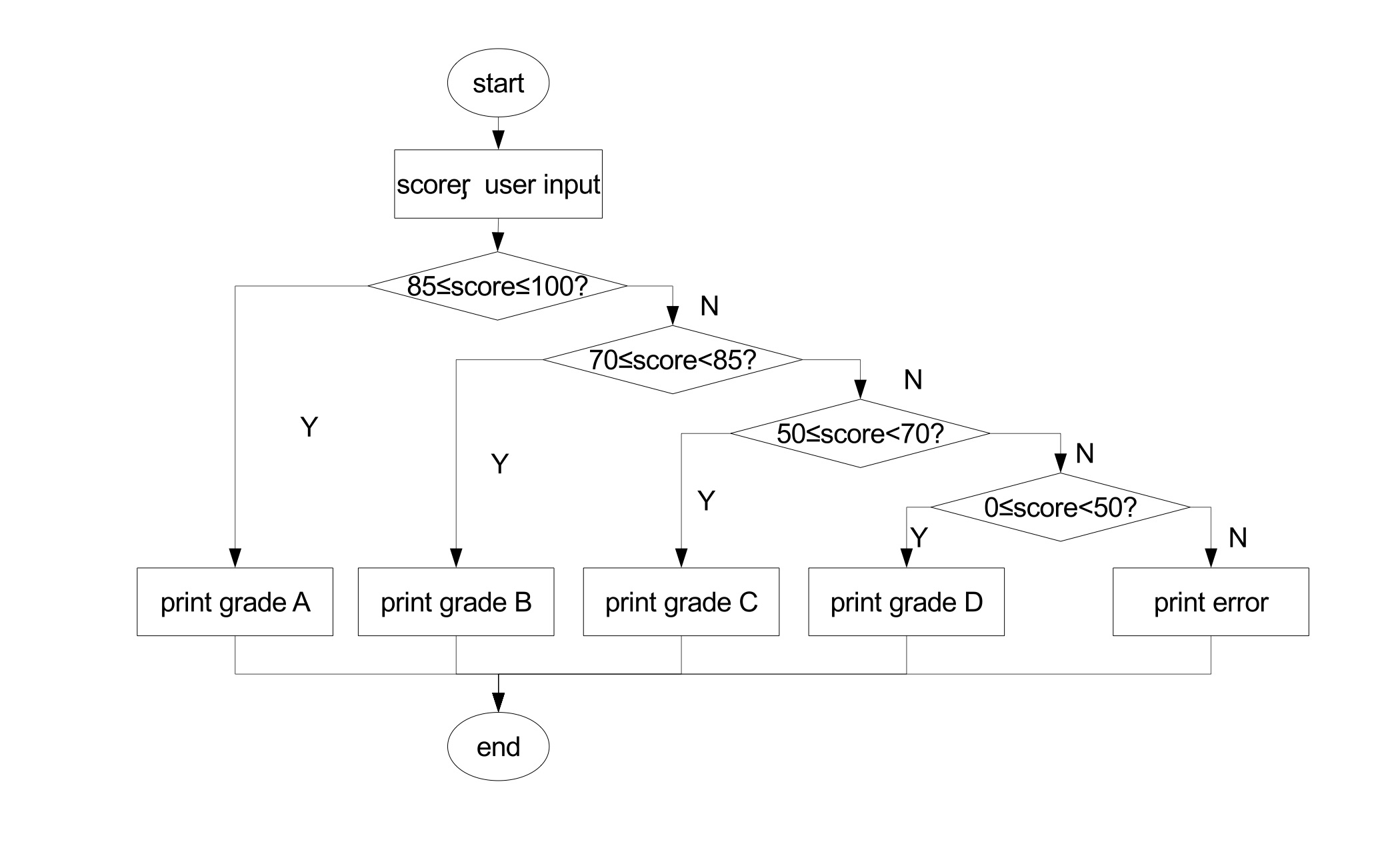
Fig. 5.4 Five-way branching example.¶
Note that the program above consists of only two top-level statements: an assignment statement and a compound if … elif … else … statement. The flow chart of a compound if … elif … else … statement is always right-branching—additional elif Boolean expressions always split the right branch from its previous branching point.
Finally, the general form of the if statement, which consists of one if component, zero or many elif components, and zero or one else component, is shown in Fig. 5.4.
Walnus operator. The Walrus Operator is a new syntax introduced in Python 3.8 that allows you to assign values to variables within an expression. This is particularly useful when you want to use the value of a variable multiple times within the same expression. The Walrus Operator is denoted by the := symbol.
The following program uses the Walrus Operator to assign the value of x + 5 to y within the if statement. If y is greater than 10, we print “y is greater than 10,” and if it’s less than or equal to 10, we print “y is less than or equal to 10.”
Check your understanding
- Just one.
- Each block may also contain more than one.
- Zero or more.
- Each block must contain at least one statement.
- One or more.
- Yes, a block must contain at least one statement and can have many statements.
- One or more, and each must contain the same number.
- The blocks may contain different numbers of statements.
5.2Q-1: How many statements can appear in each block (the if and the else) in a conditional statement?
- TRUE
- TRUE is printed by the if-block, which only executes if the conditional (in this case, 4+5 == 10) is true. In this case 5+4 is not equal to 10.
- FALSE
- Since 4+5==10 evaluates to False, Python will skip over the if block and execute the statement in the else block.
- TRUE on one line and FALSE on the next
- Python would never print both TRUE and FALSE because it will only execute one of the if-block or the else-block, but not both.
- Nothing will be printed
- Python will always execute either the if-block (if the condition is true) or the else-block (if the condition is false). It would never skip over both blocks.
5.2Q-2: What does the following code print (choose from output a, b, c or nothing).
if 4 + 5 == 10:
print("TRUE")
else:
print("FALSE")
- Output a
- Because -10 is less than 0, Python will execute the body of the if-statement here.
- Output b
- Python executes the body of the if-block as well as the statement that follows the if-block.
- Output c
- Python will also execute the statement that follows the if-block (because it is not enclosed in an else-block, but rather just a normal statement).
- It will cause an error because every if must have an else clause.
- It is valid to have an if-block without a corresponding else-block (though you cannot have an else-block without a corresponding if-block).
5.2Q-3: What does the following code print?
x = -10
if x < 0:
print("The negative number ", x, " is not valid here.")
print("This is always printed")
a.
This is always printed
b.
The negative number -10 is not valid here
This is always printed
c.
The negative number -10 is not valid here
- No
- Every else-block must have exactly one corresponding if-block. If you want to chain if-else statements together, you must use the else if construct, described in the chained conditionals section.
- Yes
- This will cause an error because the second else-block is not attached to a corresponding if-block.
5.2Q-4: Will the following code cause an error?
x = -10
if x < 0:
print("The negative number ", x, " is not valid here.")
else:
print(x, " is a positive number")
else:
print("This is always printed")
- Output a
- Although TRUE is printed after the if-else statement completes, both blocks within the if-else statement print something too. In this case, Python would have had to have skipped both blocks in the if-else statement, which it never would do.
- Output b
- Because there is a TRUE printed after the if-else statement ends, Python will always print TRUE as the last statement.
- Output c
- Python will print FALSE from within the else-block (because 5+4 does not equal 10), and then print TRUE after the if-else statement completes.
- Output d
- To print these three lines, Python would have to execute both blocks in the if-else statement, which it can never do.
5.2Q-5: What does the following code print?
if 4 + 5 == 10:
print("TRUE")
else:
print("FALSE")
print("TRUE")
a. TRUE
b.
TRUE
FALSE
c.
FALSE
TRUE
d.
TRUE
FALSE
TRUE
- I only
- You can not use a Boolean expression after an else.
- II only
- Yes, II will give the same result.
- III only
- No, III will not give the same result. The first if statement will be true, but the second will be false, so the else part will execute.
- II and III
- No, Although II is correct III will not give the same result. Try it.
- I, II, and III
- No, in I you can not have a Boolean expression after an else.
5.2Q-6: Which of I, II, and III below gives the same result as the following nested if?
# nested if-else statement
if x < 0:
print("The negative number ", x, " is not valid here.")
else:
if x > 0:
print(x, " is a positive number")
else:
print(x, " is 0")
I.
if x < 0:
print("The negative number ", x, " is not valid here.")
else x > 0:
print(x, " is a positive number")
else:
print(x, " is 0")
II.
if x < 0:
print("The negative number ", x, " is not valid here.")
elif x > 0:
print(x, " is a positive number")
else:
print(x, " is 0")
III.
if x < 0:
print("The negative number ", x, " is not valid here.")
if x > 0:
print(x, " is a positive number")
else:
print(x, " is 0")
- a
- While the value in x is less than the value in y (3 is less than 5) it is not less than the value in z (3 is not less than 2).
- b
- The value in y is not less than the value in x (5 is not less than 3).
- c
- Since the first two Boolean expressions are false the else will be executed.
5.2Q-7: What will the following code print if x = 3, y = 5, and z = 2?
if x < y and x < z:
print("a")
elif y < x and y < z:
print("b")
else:
print("c")
© Copyright 2024 GS Ng.