Chapter 5 If Statment
5.3 Nested if Statement¶
Since a compound if statement is also a statement, it can be used in a statement block inside another if statement. This form of nested if statements is sometimes useful for organizing branches of control flow with complex conditions.
For example, in a FIFA World Cup, four teams participate in the semi-finals by paying two semi-final matches separately. The winners of the two semi-final matches play a final match for the World-Cup championship, while the losers of the two semi-final matches play a match for the third place. In this process, each of the four teams will play two matches, and the results determine the team’s final rank in the World-Cup game. The following Python code simulates the game.
The program consists of three statements, the first two being assignment statements that inquire the user for the results of the two matches that a team played. The third statement is a compound if statement that contains two indented statement blocks, each consisting of another if statement. An example of executing semi_final.py is shown below.
> python semi_final.py
Did the team win the first game? [Y/N] Y
Did the team win the second game? [Y/N] N
Second place
> python semi_final.py
Did the team win the first game? [Y/N] N
Did the team win the second game? [Y/N] Y
Third place
> python semi_final.py
Did the team win the first game? [Y/N] Y
Did the team win the second game? [Y/N] Y
First place
For analyzing the dynamic behavior of nested branching statements, a hierarchical approach can be useful—the branching statement of the outer level can be analyzed first (without considering the behavior of branches in the inner level), before the branching statements in the inner level are analyzed independently. In each level, the if statement behaves exactly as introduced earlier depending only on the Boolean expression in the if line. Nesting does not change the mechanism of each individual statement. The flow chat of semi_final.py is shown in Fig. 5.5(a).
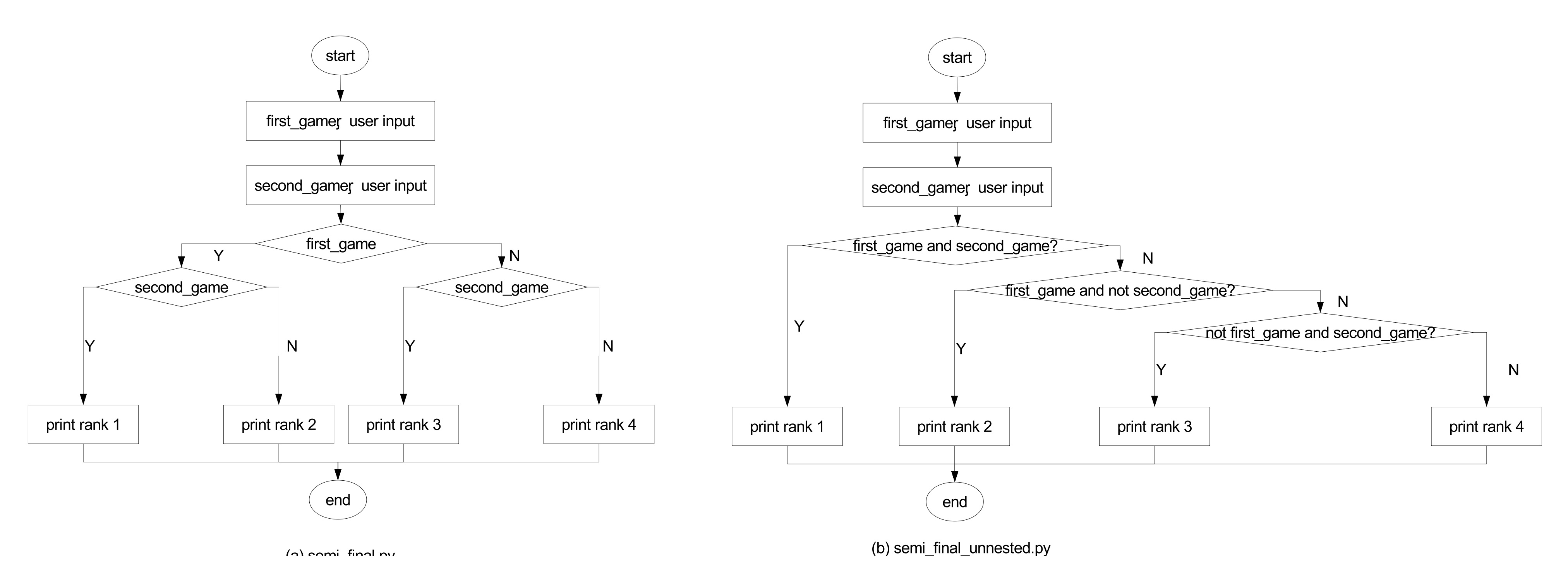
Fig. 5.5 Control flow diagrams: (a) semi_final.py. (b) semi_final_unnested.py.¶
An alternative way of writing the same program, without using nested if statements, is to use an if statement with combined Boolean conditions.
An execution of semi_final_unnested.py yields the same result as semi_final.py. However, the logic behind semi_final_unnested.py is different from that behind semi_final.py. The control flow diagram of semi_final_unnested.py is shown in Fig. 5.5(b), where the two matches are combined into one condition, and the four different outcomes are organized in a right-branching hierarchy.
As can be seen from a comparison between Fig. 5.5(a) and Fig. 5(b) by using nested if statements, multiple Boolean conditions can be organized in an arbitrary hierarchy. In this particular example, nested if offers a more balanced and perhaps more intuitive logic hierarchy when compared to the right-branching hierarchy by the compound if … elif … else … statement without using nested if statements.
In general, all multi-way branching situations that can be addressed by using if … elif … else statements can be addressed by using nested if statements also. A choice can be made according to the convenience of expressing a logical hierarchy. Sometimes if statements can be nested in more than two levels. When there are many branches and complex hierarchical logic structures, it is possible to make a mistake by writing an incorrect Boolean condition. In such situations, drawing a control flow diagram can be useful for spotting and fixing logical mistakes. In addition, debugging techniques can be used to verify the behavior of a program dynamically.
Check your understanding
- No
- This is a legal nested if-else statement. The inner if-else statement is contained completely within the body of the outer else-block.
- Yes
- This is a legal nested if-else statement. The inner if-else statement is contained completely within the body of the outer else-block.
5.3Q-1: Will the following code cause an error?
x = -10
if x < 0:
print("The negative number ", x, " is not valid here.")
else:
if x > 0:
print(x, " is a positive number")
else:
print(x," is 0")
© Copyright 2024 GS Ng.