Chapter 6 Loop Statement
6.1 While Loop¶
The syntax of the while statement is shown in Fig. 6.1. It is identical to the syntax of the if statement in Fig. 5.2, except that the keyword is changed from if to while. For the while statement, the indented statement block is also called the loop body.
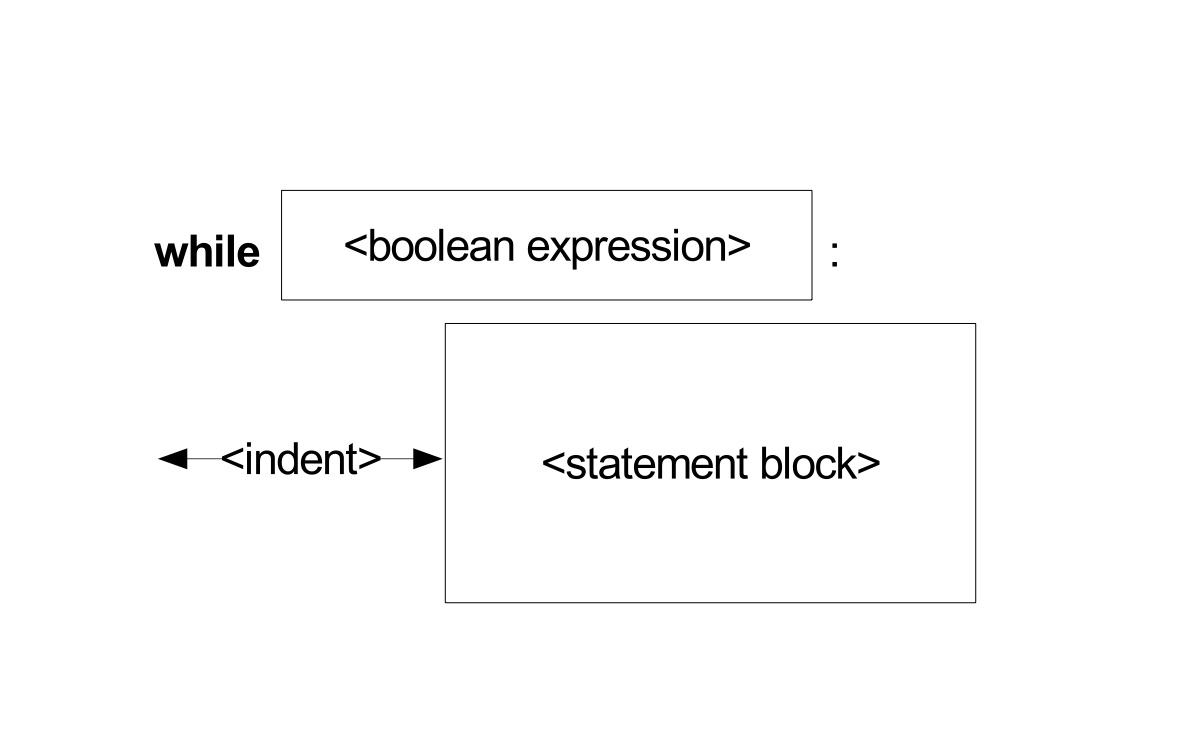
Fig. 6.1 Syntax of the while statement.¶
As shown in Fig. 6.1, the Boolean expression determines the execution of the indented statement block under the while line. If it is True, the statement block is executed; if it is False, the statement block is not executed. This is similar to the if statement. However, the while statement differs from the if statement after the execution of the indented statement block. In particular, after the execution of the indented statement block, the execution of the if statement is finished, and any further statements in the program will be executed. In contrast, after the indented statement block is executed, the while statement moves back to the Boolean expression in order to evaluate it again. If it is True this time, the indented statement block is executed again, before the Boolean expression is evaluated yet again. This process repeats (i.e. loops) as long as the value of the Boolean expression is True.
For a simple example, consider the following contrast Boolean condition:
>>> while False:
... print ('a')
>>>
In this example, the while statement does not print anything on the console, because the Boolean expression is the constant False. This is the same as with the if statement. However, when the Boolean expression is turned into True, the behavior of the while statement is different from the if statement:
>>> while True:
... print ('a')
...
a
a
a
a
a
a
a
a
a
^CTraceback (most recent call last):
File "<stdin>", line 2, in <module>
KeyboardInterrupt
a
In this example, the character ‘a’ is repeatedly printed. The execution does not stop until an external interrupt happens. In this case, the user stops the execution by using a keyboard interrupt, which can be done by pressing Ctrl-C. Keyboard interrupts can be used to stop a Python program that is currently being executed.
The infinitely repeating execution of the loop body (i.e. indented statement block in the while statement) is called an infinite loop, or a dead loop. It is one of the most common mistakes that programmers can make by not properly setting the terminating condition of the loop. In order to avoid dead loops, the value of the Boolean expression must be changed to False after a finite number of executions of the loop body. An example is shown below.
>>> i=0
>>> while i<3:
... print (i)
... i+=1
...
0
1
2
The while statement above prints the sequence of numbers 0, 1, 2 on the console. The control flow diagram of this statement is shown in Fig. 6.2(a). During dynamic execution, each statement in the loop body is executed three times, as illustrated in Fig. 6.2(b).
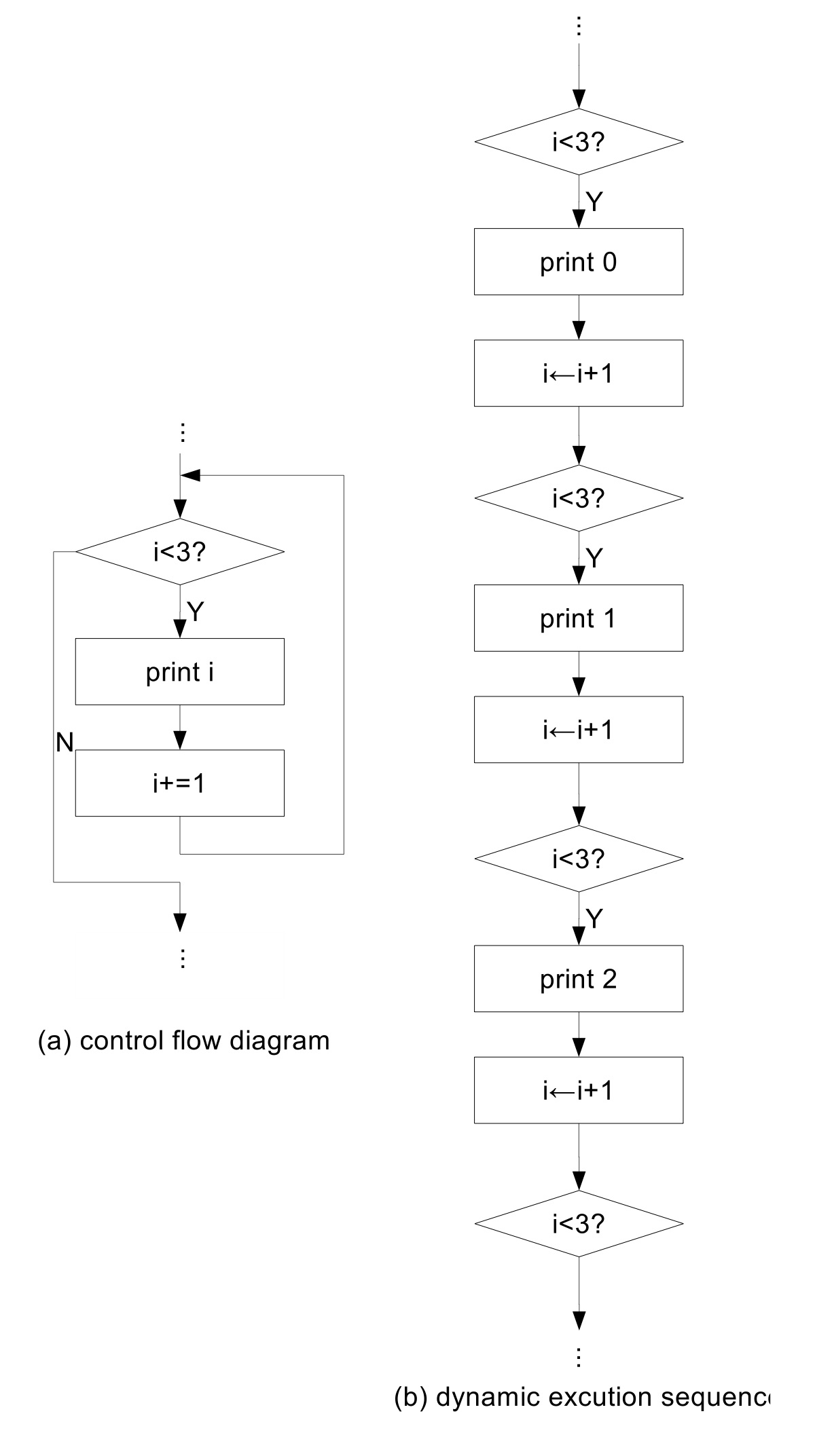
Fig. 6.2 Analysis of the dynamic behavior of a while statement: (a) control flow diagram. (b) Dynamic execution sequence.¶
The value of the variable i is set to 0 in the beginning of execution. The while statement checks the value of i in the beginning of each iteration to decide whether the loop body is executed. Inside the loop body, the value of i is incremented by 1. As a result, each time the Boolean expression i < 3 is evaluated, the value of i changes. When the value of i becomes 3 after the third iteration, the Boolean expression i < 3 becomes False and the loop finishes. It is commen to use the value of a variable (e.g. i in the example above) to control the execution of a loop, and such a variable is also called the loop variable.
Another way to control the termination of a loop is via user input. For example, consider a program that generates random letters to a user. The program works by repeatedly generating random letters to the user until she asks it to stop. This can be achieved by using a while loop, with the loop body being a random letter generator, and the looping condition being whether the user requested to stop the program.
In order to generate a random letter, one can use a random number generater together with a mapping from numbers to letters. The former is readily available in the random module, while the latter can be implemented by using a string that contains all the candidate letters. An integer can be mapped into a letter in the string by serving as the index in a getitem operation. Given this random letter generator, the full program can be written as:
The program above consists of five top-level statements. The first is an import statement for the random module. The second is an assignment statement that defines the string for mapping integers to letters. The string consists of the upper case English alphabet. The third statement is an assignment statement that requests the user to enter a command. The fourth statement is a compound while loop, which consists of a statement block that generates a random number and then asks the user for the next command. The fifth statement is a print statement that indicates the finishing of execution to the user.
The loop body consists of three statements, the first being an assignment statement that obtains a random integer that ranges over all possible indices on the string, the second being a print statement that shows the value of an expression that maps the integer to a letter by using the getitem operation, and the third being the assignment statement that asks the user for the next command.
When executed, lettergen.py repeatedly asks the user to choose from generating a random letter (‘Y’) and exiting the program (any input other than ‘Y’). Here, the user input is used in the Boolean condition, so that the value of the expression changes at each iteration.
> python lettergen.py
Generate random letter? [Y/N] Y
K
Generate random letter? [Y/N] Y
J
Generate random letter? [Y/N] Y
Z
Generate random letter? [Y/N] Y
X
Generate random letter? [Y/N] Y
G
Generate random letter? [Y/N] Y
P
Generate random letter? [Y/N] Y
W
Generate random letter? [Y/N] Y
U
Generate random letter? [Y/N] Y
V
Generate random letter? [Y/N] Y
M
Generate random letter? [Y/N] N
Bye
Check your understanding
- True
- Although the while loop uses a different syntax, it is just as powerful as a for-loop and often more flexible.
- False
- Often a for-loop is more natural and convenient for a task, but that same task can always be expressed using a while loop.
6.1Q-1: True or False: You can rewrite any for-loop as a while-loop.
- n starts at 10 and is incremented by 1 each time through the loop, so it will always be positive
- The loop will run as long as n is positive. In this case, we can see that n will never become non-positive.
- answer starts at 1 and is incremented by n each time, so it will always be positive
- While it is true that answer will always be positive, answer is not considered in the loop condition.
- You cannot compare n to 0 in while loop. You must compare it to another variable.
- It is perfectly valid to compare n to 0. Though indirectly, this is what causes the infinite loop.
- In the while loop body, we must set n to False, and this code does not do that.
- The loop condition must become False for the loop to terminate, but n by itself is not the condition in this case.
6.1Q-2: The following code contains an infinite loop. Which is the best explanation for why the loop does not terminate?
n = 10
answer = 1
while n > 0:
answer = answer + n
n = n + 1
print(answer)
- 0
- Yes, idx goes through the odd numbers starting at 1. o is at position 4 and 8.
- 1
- o is at positions 4 and 8. idx starts at 1, not 0.
- 2
- There are 2 o characters but idx does not take on the correct index values.
6.1Q-3: How many times is the letter o printed by the following statements?
s = "python rocks"
idx = 1
while idx < len(s):
if s[idx]=='o':
print(s[idx])
idx = idx + 2
Note
This workspace is provided for your convenience. You can use this activecode window to try out anything you like.
© Copyright 2024 GS Ng.